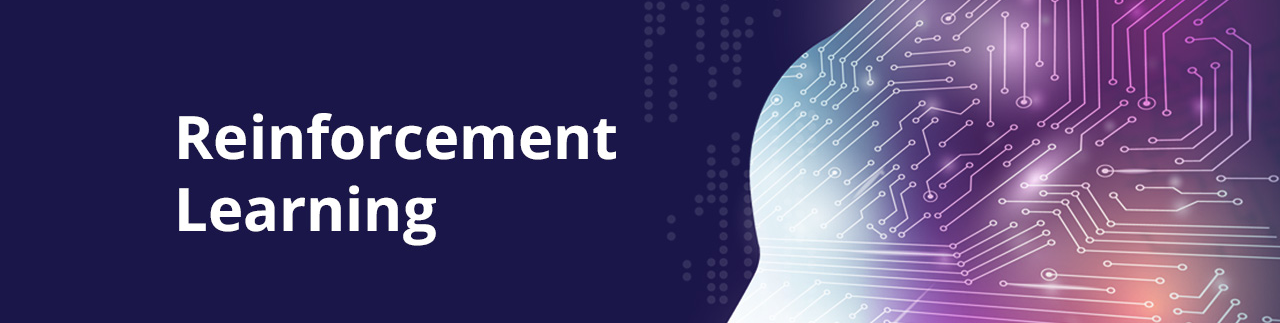
Hi everyone! It’s been a couple of weeks since my last post—life got a bit busy, and I’ll admit I got distracted along the way. But I’m back now, and I’ve been diving deep into some exciting topics: Reinforcement Learning (RL), specifically off-policy algorithms like Q-learning, and the fascinating world of attention mechanisms in Large Language Models (LLMs). I’ve also been working on an RL-based Tic-Tac-Toe game, which is nearly complete except for some tricky training hurdles. Plus, I stumbled upon an amazing video that broke down different types of attention mechanisms—more on that later! In this post, I’ll walk you through what I’ve learned, the challenges I’ve faced, and why I’m so hooked on these topics. Let’s get started!
Off-Policy RL Algorithms
Reinforcement Learning is all about teaching an agent to make smart decisions by interacting with an environment—like training a virtual pet to fetch a ball. RL algorithms come in two flavors: on-policy and off-policy. On-policy algorithms learn from the actions the agent takes based on its current strategy (or policy), while off-policy algorithms can learn from actions taken by different policies, even from past experiences or random moves. This flexibility allows off-policy methods to reuse old data effectively.
My focus has been on Q-learning, a classic off-policy algorithm. Imagine you’re playing a game and trying to figure out the best move in every situation. Q-learning builds a “Q-table” that scores each possible action in each state—like a cheat sheet telling you how valuable each move is. Over time, it updates these scores based on rewards (e.g., +1 for a win) and potential future outcomes.
Let’s break it down:
- \( s \): The current situation (state) you’re in.
- \( a \): The move (action) you choose.
- \( r \): The immediate reward you get (e.g., points for a good move).
- \( s' \): The new situation after your move.
- \( \alpha \): The learning rate—how quickly you update your cheat sheet (between 0 and 1).
- \( \gamma \): The discount factor—how much you care about future rewards (also between 0 and 1).
- \( \max_{a'} Q(s', a') \): The best possible score you could get in the next situation.
Think of it like this: you’re tweaking your strategy a little (\( \alpha \)) based on what you just earned (\( r \)) plus a sneak peek at the best future outcome, adjusted by how much you value the long game (\( \gamma \)). Q-learning is especially useful in robotics or game AI, where the agent learns optimal moves without needing a strict playbook.
Training an RL Model for Tic Tac Toe: A Step-by-Step Guide
Tic Tac Toe is a classic board game that, despite its simplicity, provides a fantastic testbed for Reinforcement Learning (RL). In this guide, we’ll walk through the detailed process of training an RL agent—using methods like Q-learning—to master Tic Tac Toe. Every step is broken down so that even if you’re new to RL, you can understand the rationale and techniques behind each decision.
1. Understanding the Environment and Game Dynamics

In Tic Tac Toe, the environment is the game board—a 3x3 grid where each cell can be empty, marked with an “X”, or an “O”. The RL agent interacts with this environment by placing its mark on the board. The game can result in a win, loss, or draw, and we assign:
- +1 reward for a win
- 0 reward for a draw
- -1 reward for a loss
2. State Representation and Q-Table Initialization
To allow our agent to learn, we first need a way to represent each state of the board. A common approach is:
- Encoding the Board: Represent the 3x3 grid as a vector or a unique number. For instance, you can assign each cell a value (e.g., 0 for empty, 1 for “X”, -1 for “O”) and then flatten the grid into an array. Alternatively, you can generate a unique key for each board configuration.
- Q-Table: The Q-table is a lookup table where each key corresponds to a state and each value is an array that holds the Q-value for each possible action (i.e., placing a mark in one of the empty cells). Initially, all Q-values are set to zero.
3. Action Selection: Balancing Exploration and Exploitation
To train effectively, the agent must both explore new moves and exploit known rewarding moves. This is typically handled by an epsilon-greedy policy:
- With probability ε (epsilon), choose a random move (exploration).
- With probability 1 - ε, select the move with the highest Q-value for the current state (exploitation).
Over time, epsilon is usually decayed so that the agent explores less and exploits more once it has gained sufficient knowledge.
4. The Q-Learning Update Rule
At the heart of training the agent is the Q-learning update rule. When the agent takes an action, it observes the resulting reward and the next state, and then updates its Q-value for the state-action pair using the formula:
Here:
- \(s\): Current state (the board configuration before the move).
- \(a\): Action taken (the move made).
- \(r\): Immediate reward (win, draw, or loss).
- \(s'\): Next state (the board after the move).
- \(\alpha\): Learning rate, determining how fast the agent updates its knowledge.
- \(\gamma\): Discount factor, indicating the importance of future rewards.
- \(\max_{a'} Q(s', a')\): The maximum predicted future reward for the next state.
This rule helps the agent refine its “cheat sheet” (the Q-table) by blending immediate feedback with its estimation of long-term benefits.
5. Training Loop and Episode Iteration
Training an RL agent for Tic Tac Toe involves running multiple episodes of the game. In each episode:
- Reset the Environment: Start with an empty board.
- Play a Game: The agent (or two agents playing against each other) makes moves until the game ends.
- Update Q-Values: After each move, update the Q-table using the Q-learning rule.
- End of Episode: Once the game concludes (win, loss, or draw), record the outcome and reset the board for the next episode.
By iterating over thousands of episodes, the agent gradually learns which moves lead to success. You can monitor the performance by periodically evaluating the win/draw/loss ratio.
6. Challenges and Considerations
Although Tic Tac Toe has a limited number of board configurations (roughly 5,478 unique states after accounting for symmetry), there are several challenges:
- State Space Redundancy: Many board states are equivalent under rotation or reflection. Recognizing these can help reduce redundant learning.
- Exploration vs. Exploitation: Finding the right balance is critical. Too much exploration can slow learning, while too much exploitation can trap the agent in suboptimal strategies.
- Hyperparameter Tuning: Choosing appropriate values for the learning rate (\(\alpha\)), discount factor (\(\gamma\)), and exploration rate (\(\epsilon\)) is essential for effective training.
7. Optimizing Training
To further enhance performance:
- Vectorized Operations: If implementing in Python, use libraries like NumPy to perform batch updates and speed up computations.
- State Hashing: Use efficient data structures to quickly retrieve and update Q-values for board states.
- Curriculum Learning: Start with simpler scenarios (e.g., fewer moves or restricted board areas) before moving to full-game training.
With these steps and considerations, you can build an RL agent that learns to play Tic Tac Toe effectively, gaining insights that can be transferred to more complex environments.
Understanding Attention Mechanisms in Deep Learning: A Detailed Guide
Attention mechanisms have revolutionized how models process sequences, allowing them to focus on the most relevant parts of the input. In this section, we break down the different types of attention—explaining their workings step by step so that the underlying mathematics and intuition become crystal clear.
A. Self-Attention .....
Self-attention is the fundamental building block behind many modern language models. It allows every element of a sequence (e.g., each word in a sentence) to interact with every other element, determining the importance of one word in the context of another.
- Input Representation: Every word in the input sequence is converted into a high-dimensional vector (embedding) that captures its semantic meaning.
-
Linear Projections: For each word, three vectors are derived using learned linear transformations:
- Query (Q): Represents the word’s request for contextual information.
- Key (K): Encodes the word’s content that might be relevant to others.
- Value (V): Contains the actual information that will be aggregated.
- Score Calculation: For a given word, compute the dot product between its query vector and the key vectors of every word in the sequence. This yields a score for each word pair, quantifying their compatibility.
- Scaling: Divide the dot products by \(\sqrt{d_k}\) (where \(d_k\) is the dimension of the key vectors) to stabilize gradients during training.
- Softmax Normalization: Apply the softmax function to the scaled scores to convert them into a probability distribution (attention weights) that sums to 1.
- Weighted Sum: Multiply each value vector by its corresponding attention weight and sum the results to obtain the final representation for the word.
\[ \text{Attention}(Q, K, V) = \text{softmax}\left(\frac{QK^T}{\sqrt{d_k}}\right) V \]
In essence, self-attention enables the model to dynamically reassemble information by highlighting which words in the sentence are most relevant to each other.
B. Multi-Head Attention .....
Multi-head attention extends self-attention by running multiple attention mechanisms, or “heads,” in parallel. This allows the model to capture different types of relationships and features from the input.
- Multiple Projections: The input embeddings are projected into multiple sets of Q, K, and V vectors—one set for each head. Each head is trained to focus on different aspects of the input.
-
Parallel Attention Computation: Each head computes attention independently using the same self-attention mechanism:
\[ \text{head}_i = \text{Attention}(QW_i^Q, KW_i^K, VW_i^V) \]
-
Concatenation and Final Projection: The outputs from all heads are concatenated and passed through a final linear layer to combine the information:
\[ \text{MultiHead}(Q, K, V) = \text{Concat}(\text{head}_1, \dots, \text{head}_h) W^O \]
By attending to different parts of the sequence in parallel, multi-head attention enriches the model’s capacity to understand complex and varied dependencies.
C. Grouped Query Attention .....
Grouped Query Attention is designed to reduce the computational overhead inherent in the full attention mechanism. Instead of processing each query independently, similar queries are grouped together, which allows the model to share computations:
- Clustering Queries: Identify queries that are similar or share common characteristics. These queries are grouped so that their attention calculations can be computed collectively.
- Shared Computation: Compute the attention scores for the grouped queries as a batch, reducing the total number of individual operations required.
- Efficiency Trade-Off: While grouping might introduce a slight approximation error, the reduction in computation significantly speeds up the process, especially beneficial for very large models.
This approach allows models to maintain high accuracy while scaling to longer sequences or larger datasets.
D. Multi Latent Attention Mechanism .....
The video blew my mind with DeepSeek’s approach—it’s reportedly 57 times faster than standard attention! How? It optimizes how queries and keys interact, possibly with sparse attention (only looking at nearby or key words) or clever matrix tricks. It’s a game-changer for real-time applications.
Here’s the video that explains it all—highly recommend checking it out:
In the evolving landscape of deep learning, attention mechanisms play a critical role in capturing complex dependencies within input sequences. DeepSeek has introduced an innovative approach known as the Multi Latent Attention Mechanism (MLAM), which leverages latent variable modeling to dynamically extract, aggregate, and fuse multiple levels of context while significantly reducing computational overhead. In this section, we delve into the technical depths of MLAM, detailing its theoretical foundations, mathematical formulation, algorithmic steps, and comparative advantages.
Theoretical Foundations and Motivation
Traditional attention mechanisms—such as self-attention and multi-head attention—tend to compute pairwise interactions between all tokens in an input sequence. While effective, these methods scale quadratically (O(n²)) with sequence length, which can become a bottleneck in processing long sequences. To overcome this, MLAM introduces latent variables that capture the underlying structure of the input data. Instead of attending to every token explicitly, the model projects the input into a lower-dimensional latent space, where key patterns and relationships are distilled into a compact representation.
Mathematical Formulation
The Multi Latent Attention Mechanism can be conceptually divided into three major stages:
-
Latent Variable Extraction: Given an input sequence represented by embeddings \( X \in \mathbb{R}^{n \times d} \) (with \( n \) tokens and dimension \( d \)), MLAM first projects these embeddings into \( L \) latent components using a set of learned linear transformations:
\[ Z = f(X; W_Z) \quad \text{where} \quad Z \in \mathbb{R}^{n \times L} \]Here, \( W_Z \) denotes the projection matrix, and \( Z \) encapsulates the latent features.
-
Latent Attention Computation: For each latent component, separate query, key, and value projections are computed. The attention scores for each latent channel are calculated by comparing the latent queries with the keys, scaled appropriately:
\[ \text{Attention}_l(Q_l, K_l, V_l) = \text{softmax}\left(\frac{Q_l K_l^T}{\sqrt{d_k}}\right) V_l \quad \text{for } l = 1, 2, \ldots, L \]Each \( Q_l, K_l, V_l \) is derived from the latent representation \( Z \) via dedicated projection matrices.
-
Aggregation and Fusion: The outputs from the \( L \) latent attention channels are then concatenated and passed through a final linear transformation to produce the final context-aware representation:
\[ \text{MLAM}(Q, K, V) = \text{Concat}(\text{Attention}_1, \ldots, \text{Attention}_L) W^O \]Here, \( W^O \) is the output weight matrix that fuses the latent attention outputs into a coherent signal.
Algorithmic Steps
- Input Projection: Transform the input embeddings \( X \) into latent space \( Z \) using a learned projection.
- Latent Splitting: For each of the \( L \) latent components, generate corresponding query (\( Q_l \)), key (\( K_l \)), and value (\( V_l \)) vectors.
- Attention Scoring: Compute the scaled dot-product attention for each latent component using the formula above.
- Aggregation: Concatenate the outputs from all latent channels.
- Final Fusion: Apply a linear transformation to integrate the aggregated attention into the final output representation.
Comparative Analysis of Attention Mechanisms
The following table compares key attributes of various attention mechanisms, highlighting the distinctive features and computational benefits of the Multi Latent Attention Mechanism.
Mechanism | Description | Computational Complexity | Key Features | Advantages | Disadvantages |
---|---|---|---|---|---|
Self-Attention | Each token attends to every other token in the sequence. | O(n²) | Global context; simplicity | Effective for moderate sequence lengths | Scales poorly with longer sequences |
Multi-Head Attention | Multiple attention heads compute parallel attention in different subspaces. | O(h * n²), with h heads | Diverse representation; rich context capture | Improved modeling capacity over single-head attention | Increased computational cost |
Grouped Query Attention | Clusters similar queries to share computations and reduce redundancy. | Below O(n²), dependent on grouping efficiency | Efficiency through query grouping | Lower computation with minor approximation | Potential loss in fine-grained detail |
Multi Latent Attention (DeepSeek) | Uses latent variable modeling to extract and aggregate multiple latent representations. | Approximately O(L * n), where L ≪ n | Dynamic latent extraction; efficient fusion; scalability | Reduces computational overhead significantly; excels with long sequences; enhanced real-time performance | Higher model complexity; requires careful tuning of latent dimensions |
Implementation Considerations
Implementing MLAM demands careful attention to several design aspects:
- Latent Dimension Selection: The number of latent components \( L \) must be balanced; too few may limit expressiveness, whereas too many can lead to overfitting and unnecessary computational burden.
- Projection Layers: Extend standard Q, K, and V projections to generate distinct latent vectors. This requires additional parameters and careful initialization.
- Normalization and Scaling: Incorporate normalization techniques such as layer normalization and appropriate scaling (e.g., division by \( \sqrt{d_k} \)) to stabilize the gradients during training.
- Fusion Strategy: The concatenation and final projection \( W^O \) must be designed to effectively merge diverse latent insights, ensuring that critical information is preserved.
Empirical Performance and Applications
Empirical studies have shown that the Multi Latent Attention Mechanism can significantly accelerate model inference and training, particularly in scenarios involving long text sequences or real-time applications. This mechanism is ideally suited for advanced language models, recommendation systems, and other tasks where efficiency and scalability are paramount.
Conclusion
DeepSeek’s Multi Latent Attention Mechanism marks a substantial leap in attention-based modeling. By incorporating latent variable modeling, MLAM not only reduces the computational complexity but also enriches the contextual representation by dynamically aggregating multiple latent factors. This approach sets the stage for the next generation of AI applications, offering unparalleled efficiency and robust performance in handling complex, real-world data.
Let's Connect & Grow Together
I'm passionate about sharing knowledge and building a community of like-minded professionals. Connect with me on these platforms and let's learn and grow together!